My C/C++ Videos on Youtube
Here is the complete playlist for video lectures and tutorials for the absolute beginners. The language has been kept simple so that anybody can easily understand them. I have avoided complex jargon in these videos.
Looping an array is to recursively process each item of the array. This process could be as simple as displaying the contents of each element.
Looping Requirements
We need to know two things for looping an array - the first is the number of elements, and the second is the mode of accessing the n-th element. The number of elements in an array can be obtained by various methods.
sizeof operator: This operator can be used if the array has been allocated on the stack, and more importantly, the array shouldn't have been received as a function parameter. The following code shows the whole concept.
int main() { int arr[10]; int arraySize = sizeof(arr); int elementSize = sizeof(int); int count = arraySize/elementSize; cout << "Total elements = " << count << endl; return 0; }
Array as a parameter: When an array is passed as a parameter, the sizeof operator won't give the correct size of your array because the compiler passes the starting address of your array as the argument. The parameter is passed in the form of a pointer. The following code shows that the things don't work on expected lines.
void fx(int arr[]) { int arraySize = sizeof(arr); // prints 4, whereas it should print more cout << "Array size(bytes) = " << arraySize << endl; } int main() { int arr[10]; fx(arr); return 0; }
The above code will give wrong results if the type of the array is later revised to a char, and the programmer doesn't make changes to the elementSize statement above. This is what I am saying:
int main() { char arr[10]; int arraySize = sizeof(arr); // DEVELOPER FORGETS THIS int elementSize = sizeof(int); int count = arraySize/elementSize; // WRONG RESULT cout << "Total elements = " << count << endl; return 0; }
The above code can be made better by using the zero-th element for obtaining the size of an element. See the changed code below. This code will work for any type of a stack allocated array.
int main() { char arr[10]; int arraySize = sizeof(arr); int elementSize = sizeof(arr[0]); int count = arraySize/elementSize; cout << "Total elements = " << count << endl; return 0; }
The above method is so general that we can think of writing a MACRO for computing the size of an array in a very general way. This is how to do it.
#define TOTAL_ELEMENTS(x) (sizeof(x) / sizeof((x)[0])) int main() { char arr[10]; int count = TOTAL_ELEMENTS( arr); cout << "Total elements = " << count << endl; return 0; }
An array can be passed by reference also, but the syntax is a bit complicated, and it requires us to pass the number of elements also. This is how we can pass an array by reference, and you can also see that the size is reported correctly.
void fx(int (&arr)[10]) { int arraySize = sizeof(arr); // prints correctly cout << "Array size(bytes) = " << arraySize << endl; } int main() { int arr[10]; fx(arr); return 0; }
The sizeof operator will not give the results correctly, even if you pass an array by value and also indicating the number of elements. In the code below we have specified the number of elements of the array, and yet the size is not reported correctly. The compiler passes an array as a pointer.
void fx(int arr[10]) { int arraySize = sizeof(arr); // prints 4, whereas it should print more cout < "array="" size(bytes)=" << arraySize << endl; } int main() { int arr[10]; fx(arr); return 0; }
When the sizeof operator is applied to a pointer, it returns the bytes occupied by an address, which is 32 on a 32-bit compiler, and 64 on a 64-bit compiler. So sizeof(void*), sizeof(int*), sizeof(any-type*) will all return 32 or 64 depending on the compilation. This should explain why the sizeof operator doesn't give correct results when it is applied to an array passed as an argument. The sizeof operator acts on the pointer, and returns 4 or 8, depending on the compiler.
The correct way of obtaining the elements inside an array parameter is to either pass it as a reference (if things allow) and use the sizeof operator, or expilcitly pass the number of elements as a second parameter to your function
n-th Element
The n-th element of an array can be accessed by using the square brackets(also called the array subscript operator) as shown below -
int main() { int arr[10]; cout << "0-th element = " << arr[0] << endl; return 0; }
The array subscript operator uses pointer arithmetic to arrive at an element at the n-th location, and then performs indirection on that address. The following shows how the 3rd element of an array can be accessed. Take note of the third statement.
int main() { int arr[10]; cout << arr[3] << endl; // same as the above statement cout << *(arr + 3) << endl; return 0; }
The array subscript operator and the associativity of addition can be used to see that the 4th statement below also gives the same results.
int main() { int arr[10]; cout << arr[3] << endl; cout << *(arr + 3) << endl; // also possible cout << 3[arr] << endl; return 0; }
Various Options for Looping
Looping an array can be done by using the for loop, while loop and do-while loops, which we have already seen. There is another loop called range based for loop which has been introduced only recently. It is similar to the foreach loop in C# and javascript. Here's the C++ code.
int main() { int x[10] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; for( int y : x ) { cout << y << " "; } return 0; }
On each iteration, the identifier y gets the values of each element one by one. For example, when the loop runs the first time, y equals 1, the next time it gets 2, and so on, till the last element has been processed. The loop stops after that.
Classroom Training
Classroom training in C/C++ is available at our training institute. Click here for details. You can also register yourself here.
Video Tutorial
We have created a video tutorial that can be downloaded and watched offline on your windows based computer. It is in the form of an exe file. No installation is required, it runs by double clicking the file. Since the exe file is hosted on the Google Drive, it should be very safe because Google itself checks for any virus or malware. The video can be watched offline, no internet is required.
Pricing
This single video will cost you USD $5. When you download the video, you will be able to watch the first few minutes for free, as a sample. The video app will prompt you for payment through PayPal and other payment options. If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials The entire set is priced at USD $50.
Screenshots
This is a screenshot of the software that should give you a good idea of the available functionality. Click on the image to see a larger view.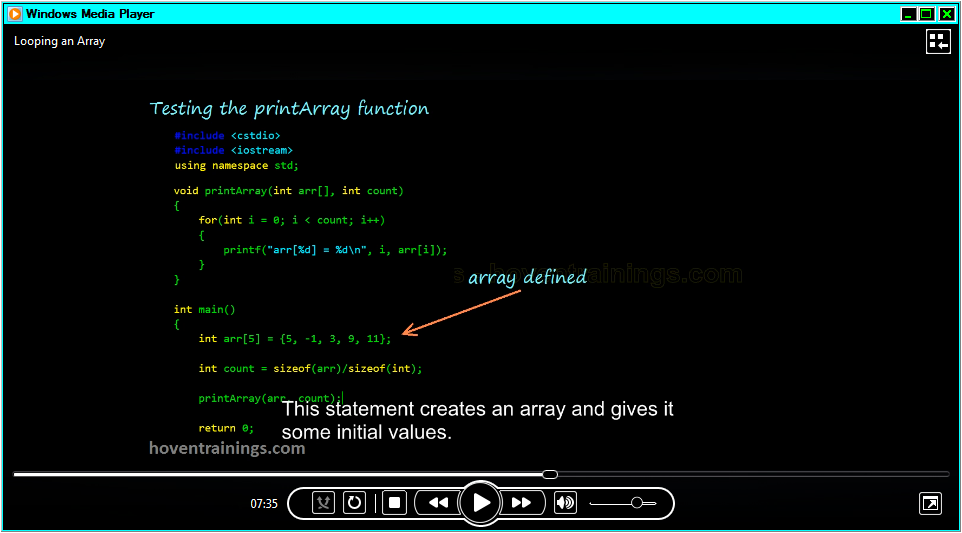
Installation
This software doesn't require any installation. Just download and double-click to run it. It doesn't require administrative priviliges to run. It runs in limited access mode, so should be very safe.
Supported Operating Systems
These videos can be run on 32-bit as well as 64-bit versions of the Microsoft Windows Operating Systems. Windows XP and all later OS are supported. If you want to run it on Windows XP and Windows Vista, then you must also have the .NET Framework Version 3.5 installed on your machines. Those users who have Windows 7 and later donot have to worry because these operating systems already have the .NET Framework installed on them.
Download Links
IMPORTANT NOTE: This download contains ONLY ONE video. The video is about the topic of this tutorial.
Want ALL Videos? If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials. TIP: If you plan to buy these videos, then it would be more economical to buy the entire set.
DISCLAIMER: Even though every care has been taken in making this software bug-free, but still please take a proper backup of your PC data before you use it. We shall not be responsible for any damages or consequential damages arising out of the use of this software. Please use it solely at your own risk.
This Blog Post/Article "Looping an Array" by Parveen (Hoven) is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License.
Updated on 2020-02-07. Published on: 2015-10-05