My C/C++ Videos on Youtube
Here is the complete playlist for video lectures and tutorials for the absolute beginners. The language has been kept simple so that anybody can easily understand them. I have avoided complex jargon in these videos.
An array is a side by side arrangement of items. Two adjacent items should share a common wall. Biscuits in a packet, and houses in a street are examples of arrays.
Arrays in C
We can create an array of 5 int by writing code like this
int arr[5];
This code declares an array of 5 int. We already know that a compiler allocates a memory address to each variable that it creates. Similarly, an array is laid at a certain memory address. But since two elements share a common wall, the elements of the array will be laid at consequetive memory locations.
Arrays can be created for any data type except functions, void and references. This is an example of creating an array for a char and float types.
char k[10]; float farr[4];
Array Initialization
Arrays can be given initial values at the time of declaration. There is a special syntax for this. This syntax is called the intializer syntax. This is how it can be done.
int arr[3] = {5, 8, 2};
An array of three int has been given initial values. These values serially go into items 1, 2 and 3 of the array. The items of an array are indexed from 0. So the first item has index of 0, the second one has an index of 1, and so on. The above items can be displayed back like this
int main() { int arr[3] = {5, 8, 2}; cout << arr[0] << endl; // prints 5 cout << arr[1] << endl; // prints 8 cout << arr[2] << endl; // prints 2 return 0; }
If the number of intializers is less than the declared size of the array, the remaining elements get default values of 0, 0.0, 0.0f or '\0' depending on the data type of the array. In the code below, the items at index 2, 3, and 4 get the value of 0, because the default value of an int is 0.
int main() { int arr[5] = {5, 8}; cout << arr[0] << endl; // prints 5 cout << arr[1] << endl; // prints 8 cout << arr[2] << endl; // prints 0 cout << arr[3] << endl; // prints 0 cout << arr[4] << endl; // prints 0 return 0; }
What happens if the number of intializers exceeds the declared size of the array? In that case the compiler gives an error, and the code doesn't compile.
// compiler error int arr[3] = {5, 8, 2, 8, 8};
Automatic initialization of an array can be done by not giving the size of the array at all. The compiler can deduce the size from the number of elements in the initializer.
// automatic intialization, no error int arr[] = {5, 8, 2, 8, 8};
Memory Allocation
It is possible to calculate the number of bytes allocated to an array by using the sizeof operator. The memory allocated to an array is also equal to the number of items in the array multiplied by the size of one element. This axoim can be used to programmatically determine the number of elements in an array.
int main() { int arr[] = {5, 8, -9, 0, 33, 274}; // prints the total allocation to arr cout << sizeof (arr) << endl; // prints the same thing cout << (6 * sizeof (int)) << endl; // number of elements in an array int totalElements = sizeof (arr) / sizeof (int); cout << "Total elements = " << totalElements << endl; return 0; }
Printing Addresses of the Elements
The array identifier contains the starting address of an array. The address can be printed by printing the array identifier. It should be cast to an int to display it as a base 10 number.
int main() { int arrI[5]; cout << "Array starts at " << (int)arrI << endl; char arrC[5]; cout << "Array starts at " << (int)arrC << endl; float arrF[5]; cout << "Array starts at " << (int)arrF << endl; return 0; }
Array address can be printed with the printf also. The format specifier %p is used to print an address. The address is printed in hexadecimal form. The specifier %d will print the same address as a base 10 decimal number.
int main() { int arrI[5]; printf("Array starts at %p\n", arrI); char arrC[5]; printf("Array starts at %p\n", arrC); float arrF[5]; printf("Array starts at %p\n", arrF); return 0; }A for loop can be used to print the addresses of the succeeding elements of an array. To print the address of the i-th element we can write &arr[i]. This expression gives the address of the i-th element of an array. And since the array elements are contiguous, we can add i to arr to obtain the address of the element at location i. Here is the code that demonstrates the whole idea.
int main() { int arrI[5]; for(int i = 0; i < 5; i++) { cout << "Address of item " << i << " = "; cout << (arrI + i) << endl; } return 0; }
Pointers and Arrays
Array addresses can be stored in a pointer variable of compatible type. If an array contains int types, then the array address can be stored in an int*. Similarly, if the array contains float, then the address can be stored in a float*.
int main() { int arrI[5]; int* p = arrI; return 0; }
Characteristics of an Array
These are the important characteristics of an array.
- Arrays have a fixed size that must be stated at the time of declaration.
- A large number of variables can be declared easily using the array syntax. It is easy to manage the variables created in this way.
- Array access is extremely fast because all the elements lie "near by".
Display elements of array
Write a program that declares an int array of 10 elements. Store the numbers 0 - 9 in the successive elements of this array, and then display them back. Use a for loop.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[10]; for (int i = 0; i < 10; i++) { arr [i] = i; } for (int i = 0; i < 10; i++) { cout << arr [i] << endl; } return 0; }
Show elements
Create a array of 5 elements and give some initial values to the elements. Then display first and last elements only.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[5] = {22,33,44,55,99}; cout << arr[0] << endl; cout << arr[4] << endl; return 0; }
Addition using an array
Write a program to add two list of 5 elements using arrays. Create two arrays and add their respective elements.#include<iostream> #include<cstdio> using namespace std; int main() { int arrOne[5] = {8, 7, 2, 0, -9}; int arrTwo[5] = {-8, -7, -2, 0, +9}; for (int i = 0; i < 5; i++) { cout << (arrOne [i] + arrTwo[i]) << endl; } return 0; }
Arrays and cube
Write a program to create an array of 5 numbers. Give any intial values. Write code to display the cube of each element of this array.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[5] = {3, 5, 7, 1, 4} ; for(int i = 0; i < 5; i++) { int cube = arr[i] * arr[i] * arr[i]; cout << "Cube of " ; cout << arr [i] << " = "; cout << cube << endl; } return 0; }
Array element location
Write a program that creates an array of 10 elements. Display the address of each element both in hexadecimal and base 10 form. You can then verify that all elements lie side by side.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[10]; for(int i = 0; i < 10; i++) { cout << "Address of element " << i << " = "; cout << (int)(arr + i) << "(base 10); "; cout << (arr + i) << "(base 16)" << endl; } return 0; }
Print address
Write a program to print the address of the first element of an array, both with and without using the & operator.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[10]; cout << "Without & operator = " << arr << endl; cout << "With & operator = " << &arr[0] << endl; return 0; }
Array addresses with a pointer
Create an int array of 5 elements and store the address of the first element in an int pointer. Then display the address of the remaining 4 elements by using pointer arithmetic on that int pointer.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[5]; int* p = arr; for(int i = 1; i < 5; i++) { cout << ++p << endl; } return 0; }
Address of variable
Write a program to display value and address of an int variable.#include<iostream> #include<cstdio> using namespace std; int main() { int var = 5; printf("Value: %d\n",var); // p is for printing an address printf("Address: %p",&var); // print address in base 10 printf("Address: %d",&var); return 0; }
Character array
Write a program to show the address of each element of a character array by using a for loop. Take array size = 4.#include<iostream> #include<cstdio> using namespace std; int main() { char c[4]; int i; for(i=0; i < 4; i++) { printf("Address of c[%d]=%p\n",i, &c[i]); } return 0; }
Swaping with pointers
Write a program to swap two numbers by using a function that takes pointer arguments.#include<iostream> #include<cstdio> using namespace std; void swap(int *a, int *b) { int temp; temp = *a; *a = *b; *b = temp; } int main() { int num1 = 5, num2 = 10; printf("Before swap num1 = %d\n",num1); printf("Before swap num2 = %d\n",num2); swap( &num1, &num2 ); printf("After swap num1 = %d\n",num1); printf("After swap num2 = %d",num2); return 0; }
Calculate sum
Create an array of 5 int and give some initial values to the elements. Write code to display the sum of the elements of this array.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[5] = {6, 8, -6, 5, 9}; int sum = 0; for(int i = 0; i < 5; i++) { sum += arr[i]; } cout << "Sum = " << sum << endl; return 0; }
Even odd
Write a program to find even or odd numbers in the list: { 3, 6, 7, 8, 11 }.#include<iostream> #include<cstdio> using namespace std; int main() { int arr[5] = {3, 6, 7, 8, 11}; for(int i = 0; i < 5; i++) { cout << (arr[i] % 2 ? "Odd: " : "Even: ") ; cout << arr[i] << endl; } return 0; }
Classroom Training
Classroom training in C/C++ is available at our training institute. Click here for details. You can also register yourself here.
Video Tutorial
We have created a video tutorial that can be downloaded and watched offline on your windows based computer. It is in the form of an exe file. No installation is required, it runs by double clicking the file. Since the exe file is hosted on the Google Drive, it should be very safe because Google itself checks for any virus or malware. The video can be watched offline, no internet is required.
Pricing
This single video will cost you USD $5. When you download the video, you will be able to watch the first few minutes for free, as a sample. The video app will prompt you for payment through PayPal and other payment options. If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials The entire set is priced at USD $50.
Screenshots
This is a screenshot of the software that should give you a good idea of the available functionality. Click on the image to see a larger view.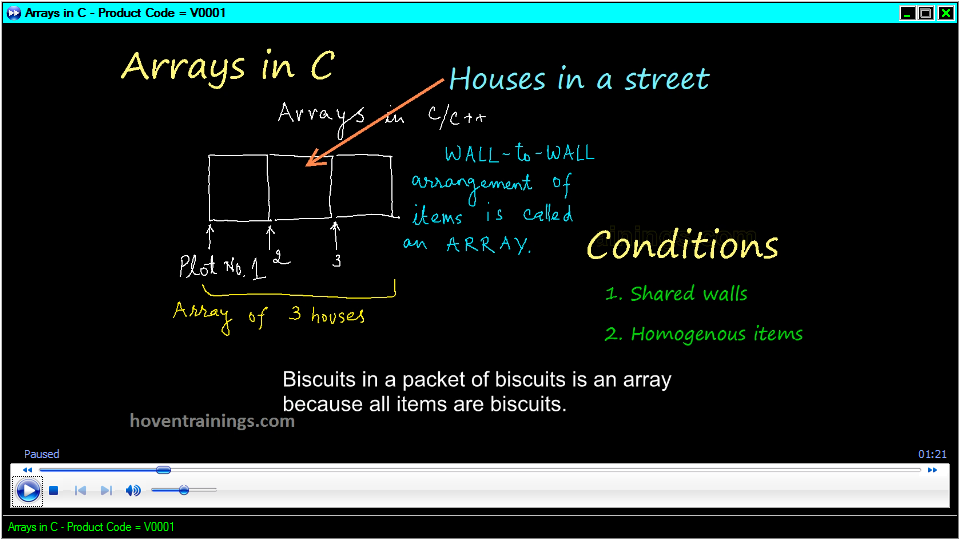
Installation
This software doesn't require any installation. Just download and double-click to run it. It doesn't require administrative priviliges to run. It runs in limited access mode, so should be very safe.
Supported Operating Systems
These videos can be run on 32-bit as well as 64-bit versions of the Microsoft Windows Operating Systems. Windows XP and all later OS are supported. If you want to run it on Windows XP and Windows Vista, then you must also have the .NET Framework Version 3.5 installed on your machines. Those users who have Windows 7 and later donot have to worry because these operating systems already have the .NET Framework installed on them.
Download Links
IMPORTANT NOTE: This download contains ONLY ONE video. The video is about the topic of this tutorial.
Want ALL Videos? If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials. TIP: If you plan to buy these videos, then it would be more economical to buy the entire set.
DISCLAIMER: Even though every care has been taken in making this software bug-free, but still please take a proper backup of your PC data before you use it. We shall not be responsible for any damages or consequential damages arising out of the use of this software. Please use it solely at your own risk.
This Blog Post/Article "Arrays in C" by Parveen (Hoven) is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License.
Updated on 2020-02-07. Published on: 2015-10-05