My C/C++ Videos on Youtube
Here is the complete playlist for video lectures and tutorials for the absolute beginners. The language has been kept simple so that anybody can easily understand them. I have avoided complex jargon in these videos.
In this tutorial I will discuss what is commonly called standard argument passing. I will slowly build my arguments to explain the whole story.
Pass by value and constancy
When an argument is passed by value, it is copied into the parameters. Any action inside the function body takes place on the parameters, which are located at a different memory location, different from those of the argument that was passed to the function. The arguments coming in, and the parameters available in the function body are two different objects, but contain the same data. You can run the following code and see that the outputs are different; different addresses are printed.
#include <iostream> using namespace std; void fx(int x) { cout << "address of x = " << &x << endl; } int main() { int x = 99; cout << "address of x = " << &x << endl; fx(x); return 0; }
This means that if you make any changes to the parameters, the changes won't have any link to the arguments that were passed in, simply because the arguments and parameters are in no way connected, being located at two different places. So, in a sense, what did our function do? It merely used the value as it's data and acted on it to produce the return value. The object was not the input - the input was the value. The function acted on the value, not the object. This is like telling somebody that cos 60° is 0.5, and then he notes it somewhere, and uses that value to perform triangulation.
The other story is also possible - the object as an input. This can be compared to a car manufacturing factory that has departments that act on the same car body, to add nuts and bolts, and finally paint it out to produce the finished product. It is possible to pass an object to a function in the manner shown below. You can run this code to verify that both the addresses are same.
#include <iostream> using namespace std; void fx(int& x) { cout << "address of x = " << &x << endl; } int main() { int x = 99; cout << "address of x = " << &x << endl; fx(x); return 0; }
The address of the argument and the parameter is the same, so they are basically one and the same object. If any changes are made to the parameter, they will have an effect on the argument being passed in.
Passing an object is a lightweight thing because no copying process is required, and no new object is required to be created. On the other hand, passing an argument by value is expensive, not only in terms of machine cycles, but also in terms of memory allocations required for holding the copies. But there is one problem when passing an object - the vulnerability. The passed object is prone to inadvertant changes in the function body. This could lead to unexpected results. This program crashes, run it and see.
#include <iostream> using namespace std; void fx(int& x) { cout << "x = " << x << endl; x -= 99; } int main() { int x = 99; int y = 99; cout << "y = " << y << endl; fx(x); // x is expected to be 99, // but the function changed it cout << "y /x should be 1" << (y / x) << endl; return 0; }
If the above function accepted its argument by value, then the results would have been on expected lines. Things go wrong when the function makes changes to the parameter, and the caller is not aware of this thing. This problem of in-advertent changes can be solved by using the const keyword with the parameters like this -
#include <iostream> using namespace std; void fx(const int& x) { cout << "x = " << x << endl; // COMPILER ERROR x -= 99; }
The compiler flags an error in this case because the incoming object comes as a const. So, if you are not going to alter the argument, and you are only going to read the value inside the function, then it is better to pass the argument through a const reference. It will ensure safety, and also prevent the overheads associated with the pass-by-value thing.
Can I use const References Always?
I see no harm in this. If you donot have to alter the incoming parameter, then passing by reference is surely the right option. But if you do have to alter the incoming parameter, then also you can obtain the input as a const reference, but create your own copy inside the function and do whatever you want to do with it. Here's a code that explains the idea.
#include <iostream> using namespace std; void fx(const int& x) { // make a local copy int z = x; z -= 99; cout << "z = " << z << endl; } int main() { int x = 99; int y = 99; cout << "y = " << y << endl; fx(x); // works fine cout << "y /x should be 1: " << (y / x) << endl; return 0; }
In either case you can make it a general rule to pass the arguments as const references. This is called standard argument passing.
The use of the const keyword speaks a lot to the user of a function. Many times the source code of a function is not available, so how will you come to know about the fate of your argument? A developer often looks for the const keyword when using a function. There are three cases which I summarize here -
- If the parameters are by value, then the caller can be sure that the arguments won't be affected.
- If the parameters are passed by reference then the caller can expect that the arguments would be altered. Now you should not think that passing by non-const is a devil. If your function uses your argument as an input-output type bi-directional parameter then it has to accept the arguments as non-const references.
- If the parameters are passed by const reference then the caller can be sure that arguments won't be altered by the calling function.
Classroom Training
Classroom training in C/C++ is available at our training institute. Click here for details. You can also register yourself here.
Video Tutorial
We have created a video tutorial that can be downloaded and watched offline on your windows based computer. It is in the form of an exe file. No installation is required, it runs by double clicking the file. Since the exe file is hosted on the Google Drive, it should be very safe because Google itself checks for any virus or malware. The video can be watched offline, no internet is required.
Pricing
This single video will cost you USD $5. When you download the video, you will be able to watch the first few minutes for free, as a sample. The video app will prompt you for payment through PayPal and other payment options. If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials The entire set is priced at USD $50.
Screenshots
This is a screenshot of the software that should give you a good idea of the available functionality. Click on the image to see a larger view.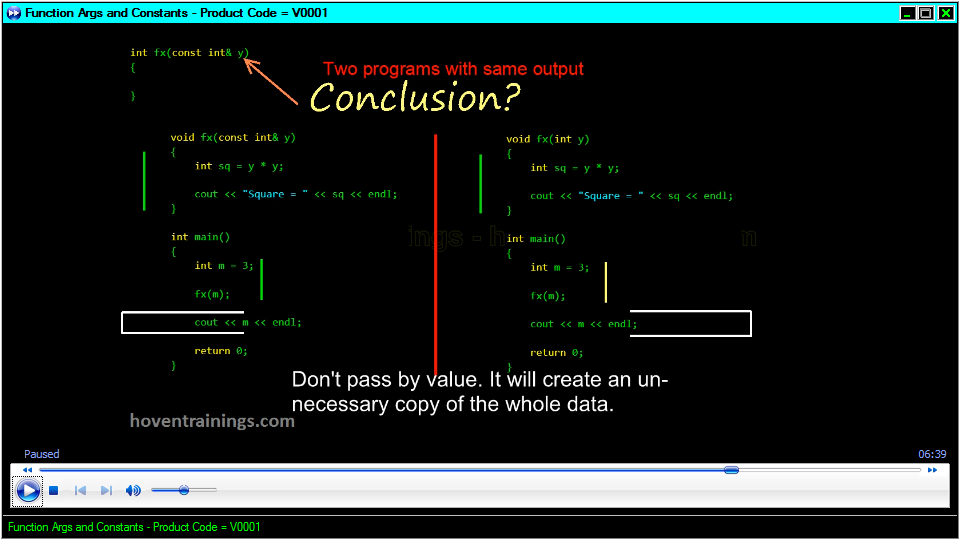
Installation
This software doesn't require any installation. Just download and double-click to run it. It doesn't require administrative priviliges to run. It runs in limited access mode, so should be very safe.
Supported Operating Systems
These videos can be run on 32-bit as well as 64-bit versions of the Microsoft Windows Operating Systems. Windows XP and all later OS are supported. If you want to run it on Windows XP and Windows Vista, then you must also have the .NET Framework Version 3.5 installed on your machines. Those users who have Windows 7 and later donot have to worry because these operating systems already have the .NET Framework installed on them.
Download Links
IMPORTANT NOTE: This download contains ONLY ONE video. The video is about the topic of this tutorial.
Want ALL Videos? If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials. TIP: If you plan to buy these videos, then it would be more economical to buy the entire set.
DISCLAIMER: Even though every care has been taken in making this software bug-free, but still please take a proper backup of your PC data before you use it. We shall not be responsible for any damages or consequential damages arising out of the use of this software. Please use it solely at your own risk.
This Blog Post/Article "Function Args and Constants" by Parveen (Hoven) is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License.
Updated on 2020-02-07. Published on: 2015-10-05