My C/C++ Videos on Youtube
Here is the complete playlist for video lectures and tutorials for the absolute beginners. The language has been kept simple so that anybody can easily understand them. I have avoided complex jargon in these videos.
int x = 8; int y = 0;
if statement
This is the syntax of an if statement. If the condition-expression is true then statement1 is executed, otherwise statement2 is executed.if(condition-expression) { statement 1; } else { statement 2; }
if can appear without else
The if part of the above statement can appear alone. An else is not compulsory.if(condition-expression) { statement 1; }But an else without a matching "if" is not possible. The compiler will flag it as an error.
Some Condition Expressions
- x == y means ( true only if x is compared equal to y )
- x != y means ( true only if x is not equal to y )
- x < y="" means="" (="" true="" only="" if="" x="" is="" less="" than="" y="">
- x > y means ( true only if x is greater than y )
- x <= y="" means="" (="" true="" only="" if="" x="" is="" less="" than="" or="" equal="" to="" y="">=>
- x >= y means ( true only if x is greater than or equal to y )
- cond1 && cond2 means (true only if both conditions are true)
- cond1 || cond2 means (false only if both conditions are false)
- !x means ( true only if x is 0 )
Ternary Operator
Following is a usage of the ternary operator. If condition is true, then y gets 7 otherwise it gets 9.int y = (condition ? 7 : 9);A ternary operator is also called an immediate if. It is useful for one-liner statements. If used judiciously, it is a very powerful operator.
Even or odd using if-else
Write a program to check whether the given number is even or odd.#include<iostream> #include<cstdio> using namespace std; int main() { int n; printf("Enter number ="); cin >> n; if(0 == n%2) { printf("no is even = %d",n); } else { printf("number is odd = %d ",n); } return 0; }
else - if
Write a program to give grade to a student according to percentage. If percentage is above 80 A grade, if percentage is between 80 - 70, than B grade . if between 70 - 60 than C grade. between 60 - 50 D grade , less than 50 is fail.#include<iostream> #include<cstdio> using namespace std; int main() { int per; printf("enter the percentage = "); cin >> per; if (per > 80) { printf ("grade A"); } else if (per > 70) { printf ("grade B"); } else if (per > 60) { printf ("grade C"); } else if (per > 50) { printf("grade D"); } else { printf("fail"); } return 0; }
if - else
write a program to check whether a person can vote or not. if person is above 18, he can vote otherwise not.#include<iostream> #include<cstdio> using namespace std; int main() { int age; cout << "Enter your age = "; cin >> age; if( age >= 18) { printf("Congrats... You can vote."); } else { printf("You can't vote...Alas..!"); } return 0; }
Pass and fail using if else
Write a program to calculate the total marks of a student in 5 subjects. than calculate percentage. If percentage is more than 40, print "pass", otherwise print "fail".#include<iostream> #include<cstdio> using namespace std; int main() { int a, b, c, d, e; printf("Enter marks for five subjects: "); cin >> a >> b >> c >> d >> e ; int sum = a + b + c + d + e; int percent = ( sum * 100 )/500; if(percent > 40) { printf("student is pass"); } else { printf("student i s fail"); } return 0; }
Use of if
Write a program using if statement. Check whether the entered number is less than 10 or not.#include<iostream> #include<cstdio> using namespace std ; int main() { int num ; cout << "Enter a number: " ; cin >> num ; if(num < 10) { cout<< "Less than 10" ; } else { cout<< "More than 10" ; } return 0 ; }
switch statement
write a program. if user enter number between 1 to 7 and get day of week#include<iostream> #include<cstdio> using namespace std; int main() { int day; cout << "Enter any no. 1 to 7"; cin >> day ; switch(day) { case 1 : cout << "Sunday"; break; case 2 : cout << "Monday"; break; case 3 : cout << "Teusday"; break; case 4 : cout << "Wednesday"; break; case 5 : cout << "Thursday"; break; case 6 : cout << "Friday"; break; case 7 : cout << "Saturday"; break; default : cout << " Wrong choice"; } return 0; }
Ternary operator
Write a program to determine whether a number is greater than 5 or not, without if-else.#include<iostream> #include<cstdio> using namespace std; int main() { printf(" Enter a number:"); int x; cin>>x; int y = ( x > 5 ? printf(" > 5 ") : printf("not > 5 ")); return 0 ; }
Ternary operator aliter
Write a program to determine whether a number is greater than 5 or not, without if-else.#include<iostream> #include<cstdio> using namespace std; int main() { cout << " Enter a number:"; int x; cin>>x; cout << "The number is: "; cout << (x > 5 ? ("") : ("not")) << endl; cout << " more than 5 " << endl; return 0 ; }
nested if-else
Write a program to check eligibility for life insurance or not. If age is less than 60, and person is physically as well as medically fit then eligible otherwise not.#include<iostream> #include<cstdio> using namespace std; int main() { int age; cout<< "Enter your age: " ; cin>> age; if (age <60) { char a; cout << "Physically Challanged?(Y/N): "; cin >> a; if(('n' == a) || ('N' == a)) { char b; cout << "Medically fit?(Y/N): "; cin >> b; if(('y' == b) || ('Y' == b)) { cout<<"Eligible"; return 0; } else { cout<< "In-eligible... medically unfit."; } } else { cout<< "In-eligible... physically unfit."; } } else { cout<< "Not eligible... Reason age."; } return 0; }
Classroom Training
Classroom training in C/C++ is available at our training institute. Click here for details. You can also register yourself here.
Video Tutorial
We have created a video tutorial that can be downloaded and watched offline on your windows based computer. It is in the form of an exe file. No installation is required, it runs by double clicking the file. Since the exe file is hosted on the Google Drive, it should be very safe because Google itself checks for any virus or malware. The video can be watched offline, no internet is required.
Pricing
This single video will cost you USD $5. When you download the video, you will be able to watch the first few minutes for free, as a sample. The video app will prompt you for payment through PayPal and other payment options. If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials The entire set is priced at USD $50.
Screenshots
This is a screenshot of the software that should give you a good idea of the available functionality. Click on the image to see a larger view.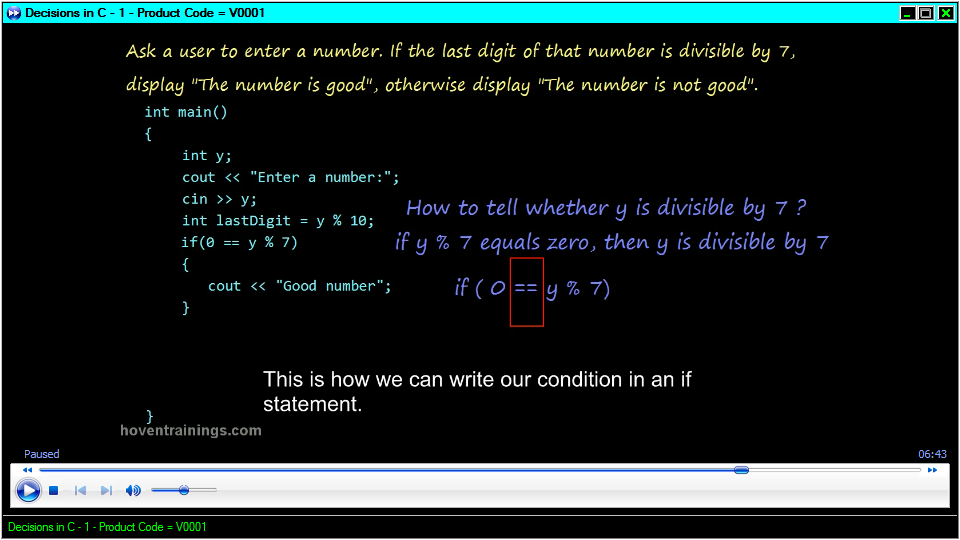
Installation
This software doesn't require any installation. Just download and double-click to run it. It doesn't require administrative priviliges to run. It runs in limited access mode, so should be very safe.
Supported Operating Systems
These videos can be run on 32-bit as well as 64-bit versions of the Microsoft Windows Operating Systems. Windows XP and all later OS are supported. If you want to run it on Windows XP and Windows Vista, then you must also have the .NET Framework Version 3.5 installed on your machines. Those users who have Windows 7 and later donot have to worry because these operating systems already have the .NET Framework installed on them.
Download Links
IMPORTANT NOTE: This download contains ONLY ONE video. The video is about the topic of this tutorial.
Want ALL Videos? If you want ALL VIDEOS, NOT JUST THIS ONE then go here: Complete Set of C/C++ Video Tutorials. TIP: If you plan to buy these videos, then it would be more economical to buy the entire set.
DISCLAIMER: Even though every care has been taken in making this software bug-free, but still please take a proper backup of your PC data before you use it. We shall not be responsible for any damages or consequential damages arising out of the use of this software. Please use it solely at your own risk.
This Blog Post/Article "If Statement in C" by Parveen (Hoven) is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License.
Updated on 2020-02-07. Published on: 2015-10-05